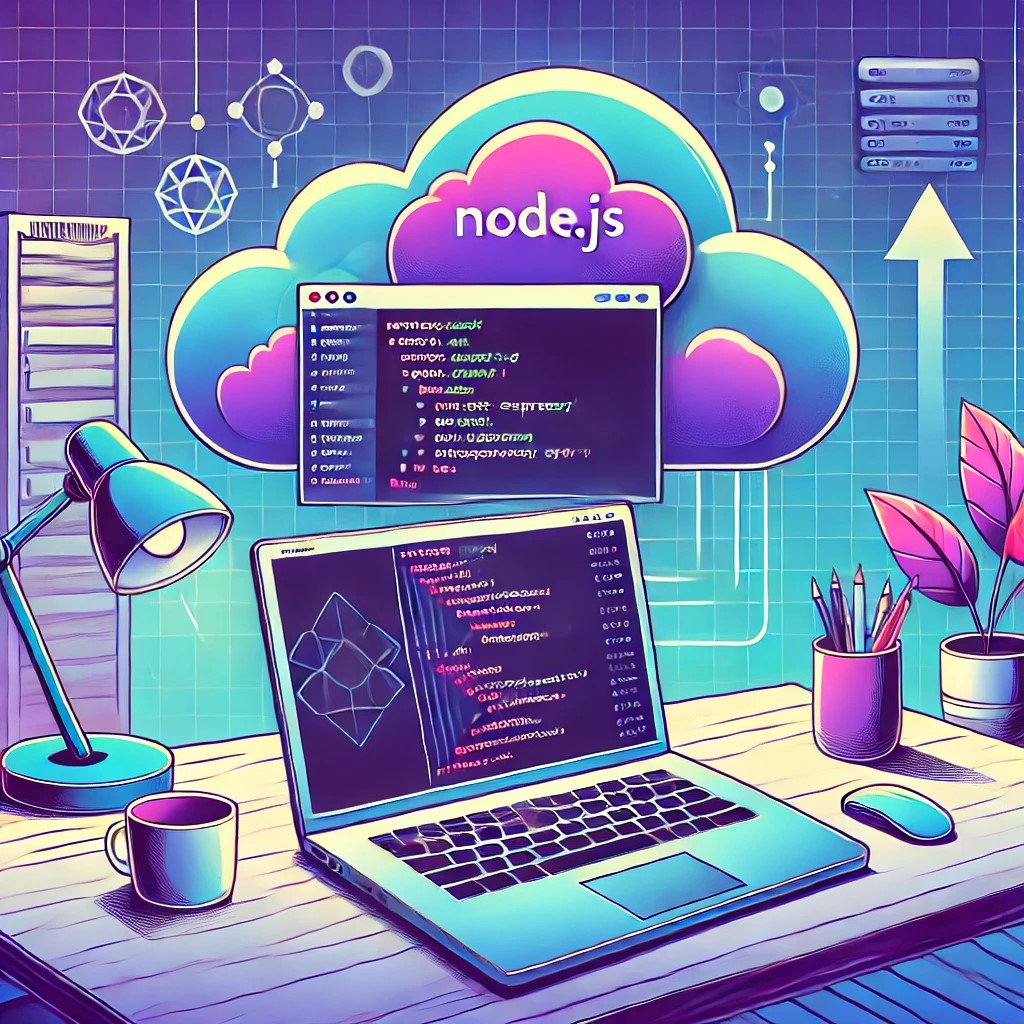
In this guide, we’ll explore how to create a simple web server using Node.js and Express, and then deploy it to Heroku. This process is ideal for beginners learning Node.js or looking to validate their skills by creating a functional project.
Setting Up the Project
Before diving into the code, ensure you have Node.js and npm installed on your system. Here’s how you can set up a basic project:
- Initialize the Project:
- Navigate to your project folder and run
npm init
. - Accept the default values by pressing
Enter
.
- Navigate to your project folder and run
- Install Express:
- Run
npm install express
to install the latest version of Express.
- Run
- Create the Project Structure:
- Organize your project with a folder structure. For example, create a folder named
src
to store your files. - Inside the
src
folder, create a file namedapp.js
.
- Organize your project with a folder structure. For example, create a folder named
Writing the Code
To start, we’ll use the "Hello World" example from the Express.js website. Follow these steps:
Open the
app.js
file and paste the following code:const express = require('express'); const app = express(); const port = process.env.PORT || 3000; app.get('/', (req, res) => { res.send('Hello World!'); }); app.listen(port, () => { console.log(`Example app listening at http://localhost:${port}`); });
- Save the file. This code sets up a simple web server that responds with "Hello World!" at the root path (
/
). - Run the server locally:
- Use the command
node src/app.js
. - Visit
http://localhost:3000
in your browser to see your app in action.
- Use the command
Adding More Features
Let’s extend the app by adding additional routes:
Update the
app.js
file:app.get('/contact', (req, res) => { res.send('Contact Us'); }); app.get('/about', (req, res) => { res.send('About Us'); });
- Restart the server and test the new paths by visiting
/contact
and/about
in your browser.
For better development efficiency, install nodemon
globally:
- Run
npm install -g nodemon
. - Start the server with
nodemon src/app.js
to automatically reload changes.
Deploying to Heroku
Once your app is running locally, deploy it to Heroku. Follow these steps:
- Set Up Heroku CLI:
- Install the Heroku CLI from heroku.com.
- Log in using
heroku login
.
- Prepare for Deployment:
- Initialize Git in your project folder (if not already done):
git init
. - Add a Heroku remote:
heroku git:remote -a <your-app-name>
.
- Initialize Git in your project folder (if not already done):
- Add Heroku-Specific Code:
- Modify the
port
variable inapp.js
to useprocess.env.PORT
. - Ensure your project includes a
Procfile
with the following content:web: node src/app.js
- Modify the
- Deploy the App:
- Commit your changes:
git add . git commit -m "Initial commit"
- Push to Heroku:
git push heroku master
- Commit your changes:
- Test the Deployment:
- Heroku provides a unique URL for your app. Visit it to ensure your app is running.
Conclusion
This guide covered the basics of creating a Node.js web server with Express and deploying it to Heroku. While this project is simple, it lays the foundation for more complex applications. As you continue learning, consider adding custom HTML pages, integrating databases, or exploring advanced features of Heroku.
Remember, learning is a continuous journey. Validate your knowledge by building projects and sharing your progress. It’s never too late to start—what matters is staying motivated and consistent.
Video Link :https://www.ravisagar.in/videos/create-basic-website-express-and-deploy-it-heroku